Using TAU – Text blocks, Locales, Numbers & Math
By Adenike Adekola / January 20, 2024 / No Comments / Checking sub-range in the range from 0 to length, Exams of Java, Java Certifications, Returning an identity string
28. Using TAU
What is TAU? Short answer: Is a Greek letter.Long answer: Is a Greek letter used to define the proportion of the circumference of a circle to its radius. Simpler, TAU is one turn of the entire circle, so 2*PI.TAU allows us to express sinuses, cosines, and angles in a more intuitive and simple way. For instance, the well-known angles of 300, 450, 900, and so on can be easily expressed in radians via TAU as a fraction of the circle, as in the following figure:
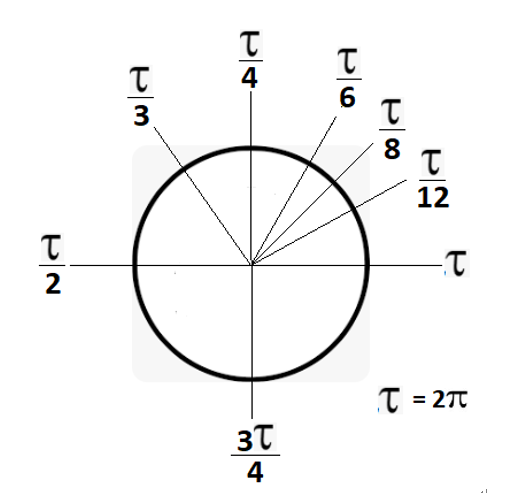
Figure 1.25 – Angles represented using TAU
This is more intuitive than PI. Is like slicing a pie into equal parts. For instance, if we slice at TAU/8 (450) it means that we sliced the pie in 8 equal parts. If we slice at TAU/4 (900) it means that we sliced the pie in 4 equal parts.The value of TAU is 6.283185307179586 = 2 * 3.141592653589793. So, the relationship between TAU and PI is TAU=2*PI. In Java, the well-known PI is represented via the Math.PI constant. Starting with JDK 19, the Math class was enriched with the Math.TAU constant.Let’s consider the following simple problem: A circle has a circumference of 21.33 cm. What is the radius of the circle?We know that: C = 2*PI*r, where C is the circumference and r is the radius. Therefore, r = C/(2*PI), or r = C/TAU. In code lines, we have:
// before JDK 19, using PI
double r = 21.33 / (2 * Math.PI);
// starting with JDK 19, using TAU
double r = 21.33 / Math.TAU;
Both approaches return a radius equal to 3.394.
29. Selecting a pseudo-random number generator
When we flip a coin or roll the dice, we say that we see “true” or “natural” randomness at work. Even so, there are tools that pretend that are capable to predict the path of flipping a coin, rolling the dice, or spinning the roulette, especially if some contextual conditions are met.Computers can generate random numbers using algorithms via the so-called random generators. Since algorithms are involved, the generated numbers are considered pseudo-random. This is known as “pseudo” randomness. Obviously, pseudo-random numbers are also predictable. How so?A pseudo-random generator starts its job by seeding data. This is the generator’s secret (the seed) and it represents a piece of data used as the starting point to generate pseudo-random numbers. If we know how the algorithm works and what the seed was then the output is predictable. Without knowing the seed the rate of predictability is very low. So, choosing the proper seed is a major step for every pseudo-random generator.Until JDK 17, Java’s API for generating pseudo-random numbers is a bit blurred. Basically, we have a robust API wrapped in the well-known java.util.Random class, and two subclasses of Random: SecureRandom (cryptographically pseudo-random generator) and ThreadLocalRandom (not a thread-safe pseudo-random generator). From a performance perspective, the relationship between these pseudo-random generators is: SecureRandom is slower than Random which is slower than ThreadLocalRandom.Next to these classes, we have SplittableRandom. This is a non-thread-safe pseudo-generator capable to spin a new SplittableRandom at each call of its split() method. This way, each thread (for instance, in a fork/join architecture) can use its own SplittableGenerator.Until JDK 17, the class hierarchy of pseudo-random generators is as in the following figure:
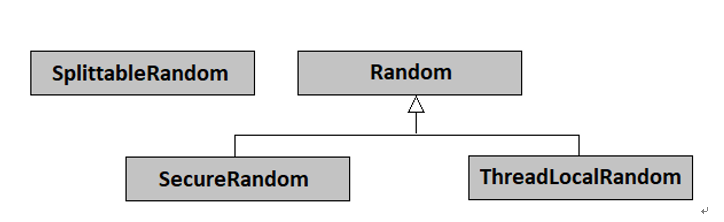
Figure 1.26 – The class hierarchy of Java pseudo-random generators before JDK 17
As this architecture reveals, changing between pseudo-random generators or choosing between different types of algorithms is really cumbersome. Look at that SplittableRandom – is lost in a no man’s land.Starting with JDK 17, we have a more flexible and powerful API for generating pseudo-random numbers. This is an interface-based API (released with JEP 356) that orbits the new RandomGenerator interface. Here is the enhanced class hierarchy of JDK 17:
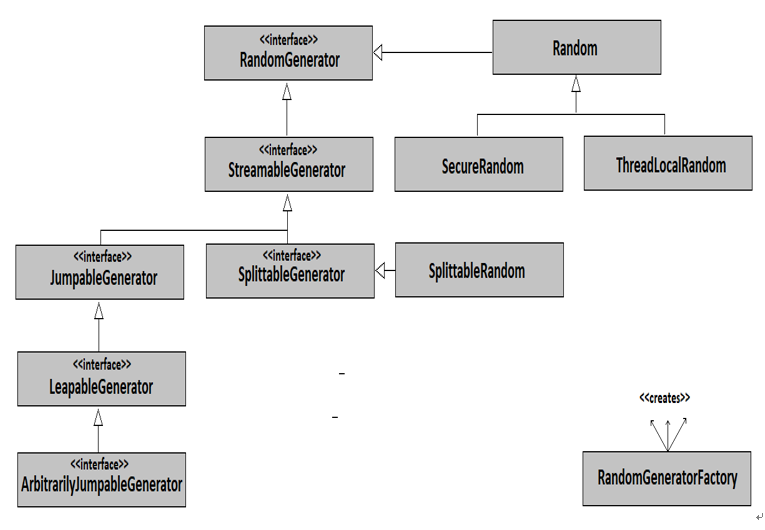
Figure 1.27 – The class hierarchy of Java pseudo-random generators starting with JDK 17
The RandomGenerator interface represents the climax of this API. It represents a common and uniform protocol for generating pseudo-random numbers. This interface has taken over the Random API and added a few more.The RandomGenerator interface is extended by 5 subinterfaces meant to provide special protocols for 5 different types of pseudo-random generators.