Rounding a float number to specified decimals – Text blocks, Locales, Numbers & Math
By Adenike Adekola / February 26, 2024 / No Comments / Checking sub-range in the range from 0 to length, Exams of Java, Filling a long array with pseudo-random numbers, Java Certifications
26. Rounding a float number to specified decimals
Consider the following float number and the number of decimals that we want to keep:
float v = 14.9877655f;
int d = 5;
So, the expected result after rounding is 14.98777.We can solve this problem straightforwardly in the least three ways. For instance, we can rely on the BigDecimal API as follows:
public static float roundToDecimals(float v, int decimals) {
BigDecimal bd = new BigDecimal(Float.toString(v));
bd = bd.setScale(decimals, RoundingMode.HALF_UP);
return bd.floatValue();
}
First, we create a BigDecimal number from the given float. Second, we scale this BigDecimal to the desired number of decimals. Finally, we return the new float value.Another approach can rely on DecimalFormat as follows
public static float roundToDecimals(float v, int decimals) {
DecimalFormat df = new DecimalFormat();
df.setMaximumFractionDigits(decimals);
return Float.parseFloat(df.format(v));
}
We define the format via setMaximumFractionDigits() and simply use this format on the given float. The returned String is passed through Float.parseFloat() to obtain the final float.Finally, we can apply a more esoteric but self-explanatory approach as follows:
public static float roundToDecimals(float v, int decimals) {
int factor = Integer.parseInt(
“1”.concat(“0”.repeat(decimals)));
return (float) Math.round(v * factor) / factor;
}
You can practice these examples in the bundled code. Feel free to add your own solutions.
27. Multiply two integers without using loops, multiplication, bitwise, division, and operators
The solution to this problem can start from the following algebraic formula also known as the special binomial product formula:
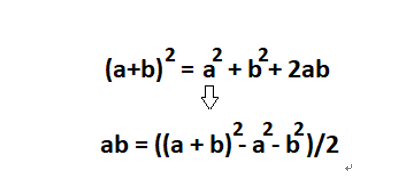
Figure 1.24 – Extracting a*b from binomial formula
Now, that we have the a*b product, there is only one issue left. The formula of a*b contains a division by 2, and we are not allowed to explicitly use the division operation. But the division operation can be mocked in a recursive fashion as follows:
private static int divideByTwo(int d) {
if (d < 2) {
return 0;
}
return 1 + divideByTwo(d – 2);
}
Nothing can stop us now from using this recursive code to implement a*b as follows:
public static int multiply(int p, int q) {
// p * 0 = 0, 0 * q = 0
if (p == 0 || q == 0) {
return 0;
}
int pqSquare = (int) Math.pow(p + q, 2);
int pSquare = (int) Math.pow(p, 2);
int qSquare = (int) Math.pow(q, 2);
int squareResult = pqSquare – pSquare – qSquare;
int result;
if (squareResult >= 0) {
result = divideByTwo(squareResult);
} else {
result = 0 – divideByTwo(Math.abs(squareResult));
}
return result;
}
In the bundled code, you can also practice a recursive approach to this problem.